My Subaru dealership’s website(s)
My Subaru Outback is due for service. Time to schedule an appointment. This is the kind of thing I just love to do online. No human interaction necessary!
Unfortunately, my dealership (who shall remain nameless, although I am happy to tell you that their shoddy website experience, which I am about to detail for you, was delivered by auto dealership web development behemoth Dealer.com) has not made the online scheduling option easy. And by “not easy,” I mean “practically impossible.”
First of all, for no good reason, the scheduling interface is built 100% in Flash. I’m pretty sure this scheduling system predates the iPhone, and Flash was at the time a de facto standard, but I’ve been building web applications since 1999 and there was never a time when it made sense to build a system like this in Flash. I deliberately don’t have Flash installed, except the version built into Chrome, so I couldn’t even load it in my browser of choice (Safari). Strike one.
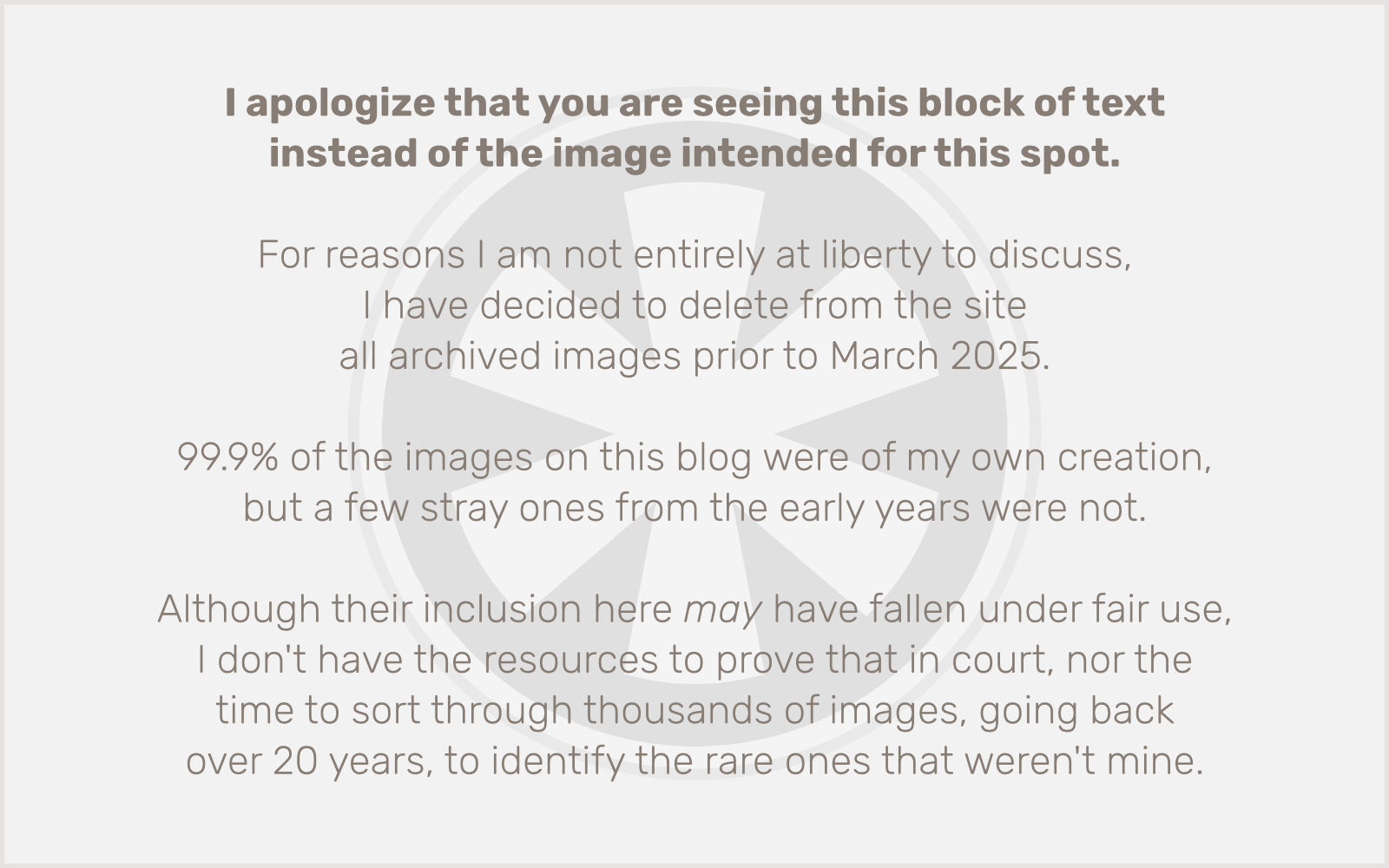
I don’t think so.
Switching over to Chrome, I was able to go through most of the appointment scheduling process, picking the service I needed done, etc., until I got to the point of actually scheduling it, where I was stuck in an endless loop of picking days that were marked “available” on a little calendar overlay, only to get an error message stating “Not available due to shop capacity.” Over and over.
All but giving up, I spotted a curious item at the bottom of the page:
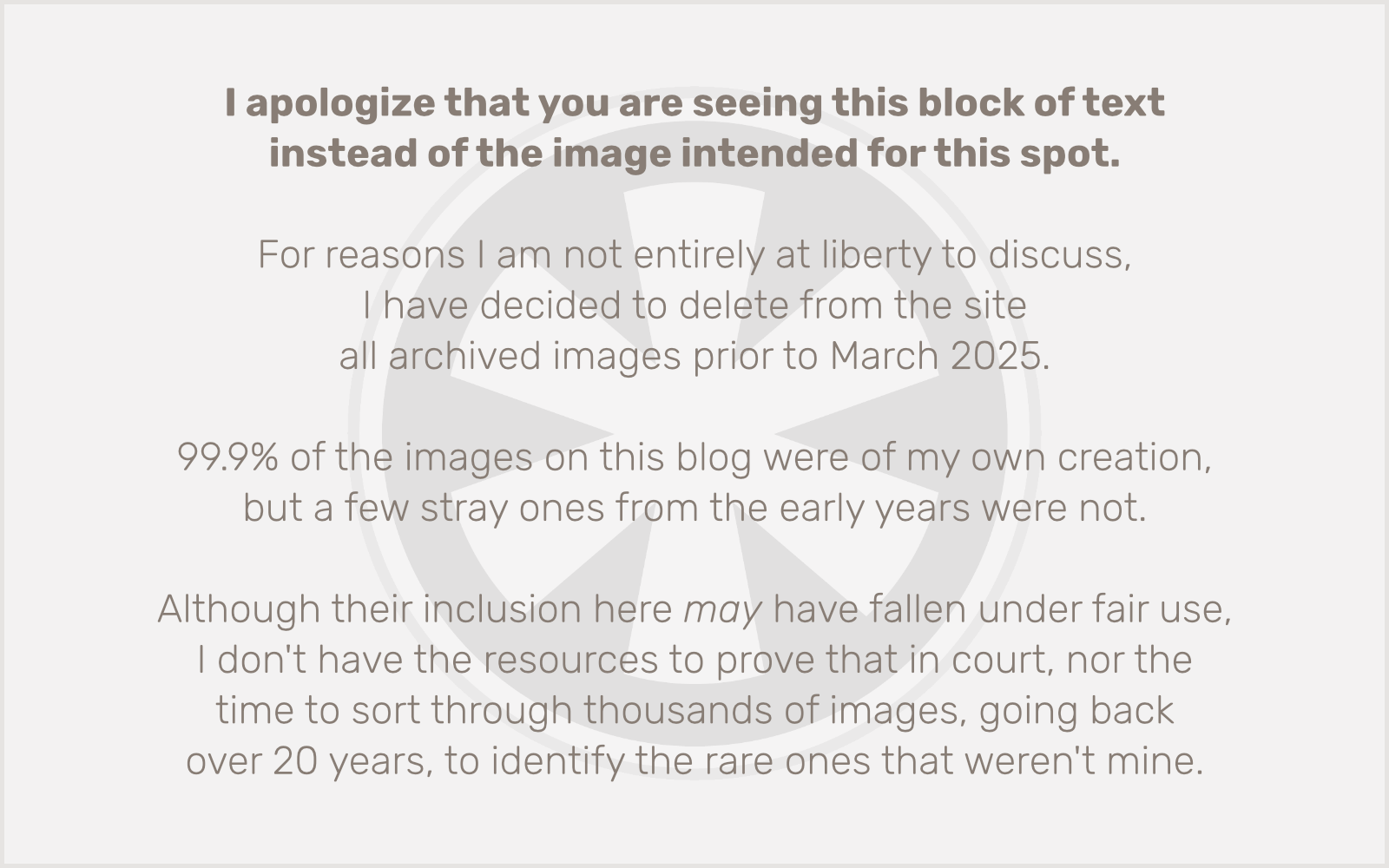
Ooh, this looks promising! Except… wait. How can clicking a link on my computer download an app to my smartphone? Maybe I should visit this site on my iPhone and then tap the link. Except… wait again. This part of the interface is in Flash, so I wouldn’t even see the link on my iPhone. So, what happens if I click it, anyway?
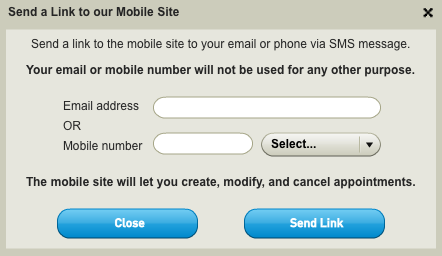
Are you kidding me? We are now at a place where I am interacting with a Flash-based web app on my computer, having it send to my phone an SMS message containing a link to the dealership’s mobile website. I’d even prefer a QR code over this convoluted mess. Strike two.
This is not how you do the Internet.
So I just decided to go over to my iPhone and try loading their website directly. It “smartly” (using the term loosely) redirected automatically to a separate iPhone-optimized version of the site, which I can only assume must be the mobile site the SMS would have linked to. (That is a reasonable assumption, right? We shall see.)
Here’s what I found when I navigated through the iPhone-optimized site to the service department’s page:
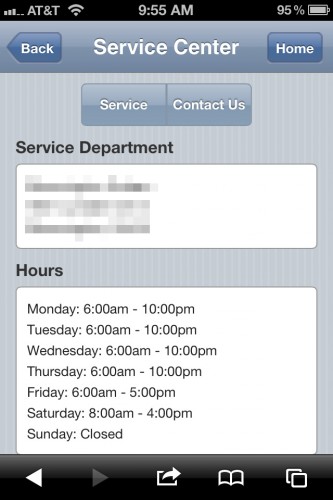
That “Service” button calls the service department. The “Contact Us” button is a link to a general-purpose email form. There are no other options.
“The mobile site will let you create, modify, and cancel appointments.” That’s what the SMS form promised. I decided (solely for the purpose of this blog post at this point) to fill out that form, but have the link emailed to me rather than sent as an SMS message. Not surprisingly, the email ended up in my junk folder. Also not surprisingly, the link goes to a different site than the version my iPhone was automatically redirected to when I tried to load the dealership’s main website. (So this is three separate websites they have now.)
I loaded this other mobile site on my iPhone, and it works!
Except, no, wait… it doesn’t:
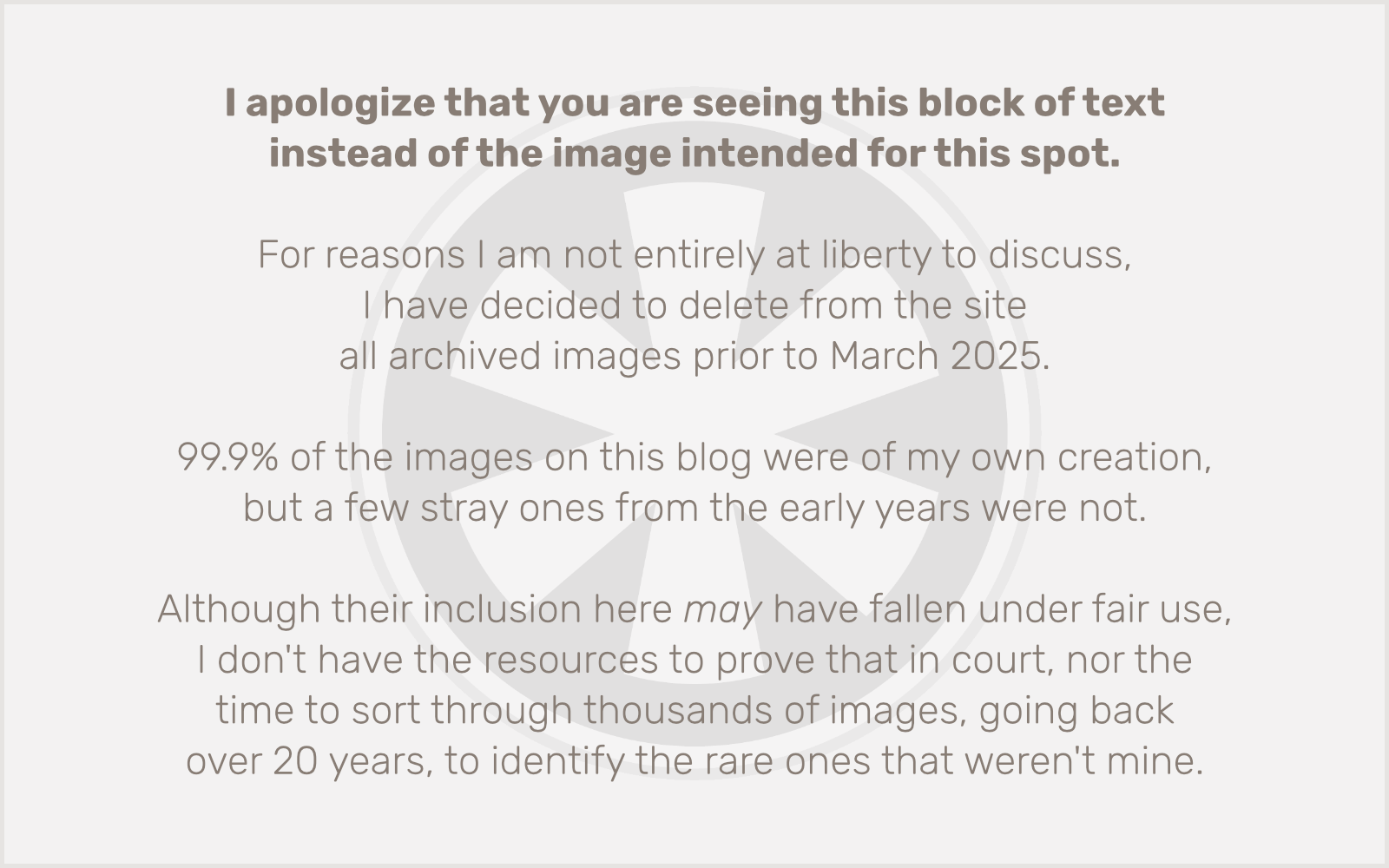
Except, no, wait again… despite that weird error message, it actually does work:
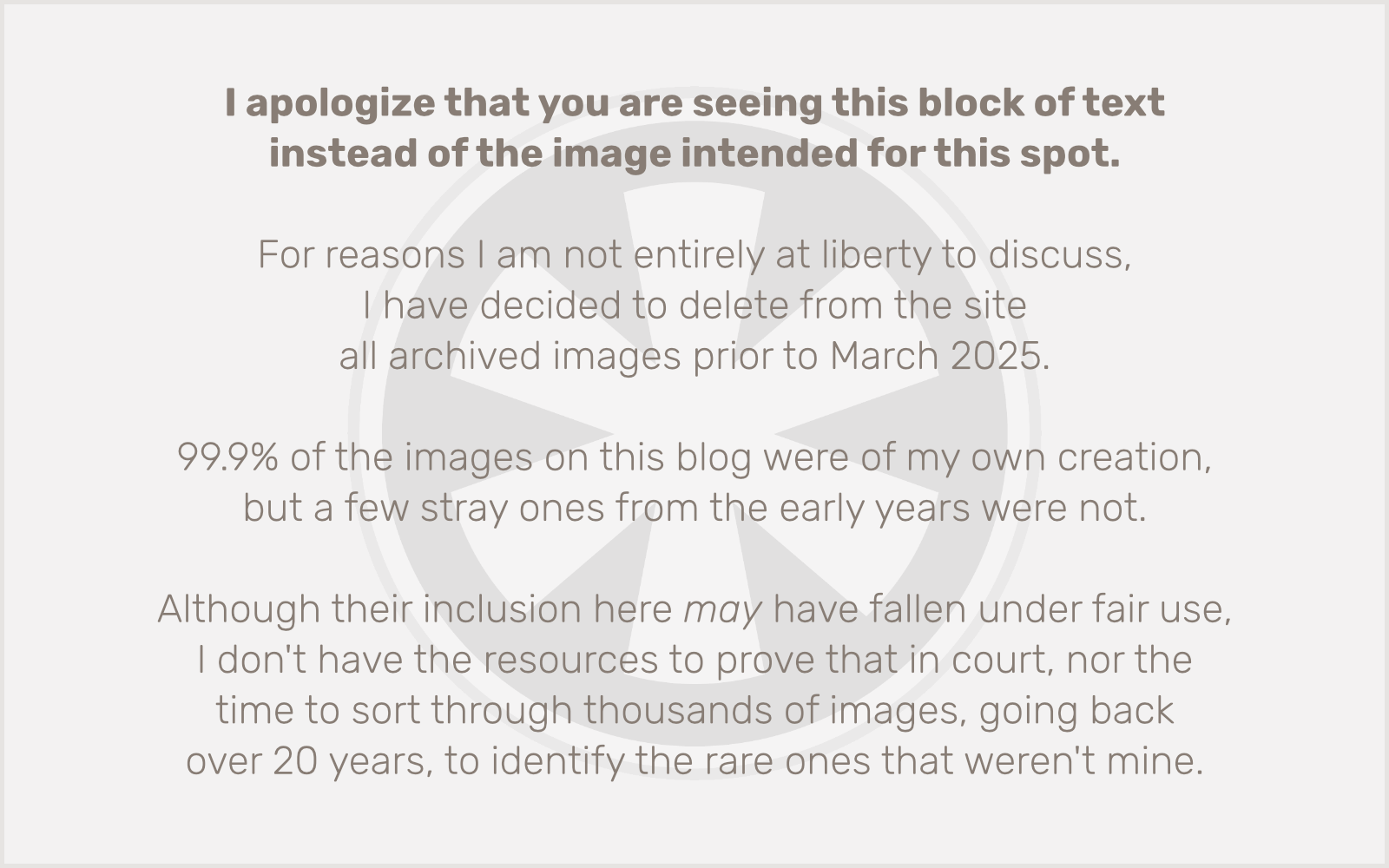
(How many “except waits” is that now?)
But since it apparently only lets you edit existing appointments, not make new ones, I guess it really doesn’t work, after all. Also, don’t ask me why the iPhone version of the site doesn’t even have a link over to this version, but I guess ultimately it doesn’t matter anyway. Strike three. And four, five and six. In fact, let’s just call the game. And I’ll call the dealership.
Conclusions
What have we learned here? Aside from the fact that I am willing to spend far more time writing a blog rant about this problem than I am just calling to schedule an appointment over the phone, I hope I have impressed the value of web standards. If this site’s interface had been built in simple HTML and JavaScript, instead of Flash, which I can tell you is 100% possible — there is absolutely nothing in the interface functionally, and not even really anything in terms of the design or interaction, that could not have been done with HTML, CSS and JavaScript, even several years ago — then it would have worked fine in Safari without Flash, and it would work on my iPhone.
There has always been a lot of temptation to use Flash to do things that couldn’t be done with web standards, especially when the standards weren’t up to Flash’s capabilities. But succumbing to that temptation led to two trends that were profoundly harmful to the open web, and the effects of which we are — and I am literally — still experiencing today.
Bad UX as standard practice
First, since the sky is the limit when designing Flash interfaces, it created a feedback loop where designers took excessive liberties in experimenting with how basic interface elements like scrollbars and buttons should look and function. Then, project stakeholders who didn’t have the requisite background to make informed UX design decisions made demands that further pushed how these elements look and function. And since Flash had so few constraints on interface design, designers could accommodate those expectations, and the situation spiraled out of control.
This problem is not exclusive to Flash, of course. These days CSS and JavaScript offer tremendous potential for messing with standard UX conventions, and I’ve gotten myself into trouble with this on occasion. Customized scrollbars, fixed-position content, etc. Always remember, just because you can do something doesn’t mean you should. Things in the future will change in ways you can’t anticipate. Those changes are likely to accommodate existing standards, but not your customizations, no matter how brilliant they may be.
Flash as an industry
Second, as Flash grew in popularity it created an entire industry of Flash developers. Suddenly you had people whose livelihood depended upon building interfaces in Flash, regardless of whether or not Flash was really the right tool for the job. And as someone who has always favored open web standards, my perspective is that Flash is only the right tool for the job when it’s the only tool for the job. Even if standards couldn’t do something in quite as… ah-hem… “flashy” a way, if they could do it at all they should be chosen over Flash. I recognize this is a bit subjective, but one thing I can say about it with objectivity is that favoring web standards over Flash for a system like the one I’m describing here would almost certainly have obviated all of the problems I encountered this morning.
A metaphorical lesson to all technology workers: when your only tool is a hammer, every problem looks like a nail. Diversify your toolbox. It will serve your clients better by ensuring that you’re using the best tool for the job, not just the tool you know best. And it will serve your career better by not limiting your job options.
Future-proofing the web
There’s an even bigger reason now that having built this kind of system then using web standards instead of Flash would’ve been a good idea. If this site’s forms had been built with semantic HTML, it would be a comparatively trivial task to build new CSS with responsive web design techniques, allowing a single site to deliver the complete user experience on any device, rather than having to pay for and build three separate, wholly inconsistent, and all inadequate websites. By supporting and adhering to standards in the past, we could (and did) build websites that still work fine today. And by continuing to adhere to standards today, we can build websites that will still work fine tomorrow, even if we don’t really know what tomorrow’s devices will look like, or how we will interact with them.