OK, I’m going to give this “liveblog” thing a shot. I’ll be posting timely updates here as I think of them, trapping every fleeting thought in amber and preserving it for subsequent humiliation. Don’t miss it! The page will automatically refresh every 5 minutes to annoy you keep you up-to-the-minute with the nonstop excitement that is bound to ensue.
11:37 PM ▸ They’re already talking automatic recount on the Senate race. Maybe it’s time for bed. For those of you who’ve hung in there with me, thanks for reading! Yes we can! And we did!
11:33 PM ▸ Al believes he’s going to win. I’m shocked. But no matter what happens, he says, “Know this: we have changed this country.”
11:30 PM ▸ Has Al Franken been drinking? I suppose that’s OK, we’re all celebrating our new president-elect. I met Al in person at the Minnesota State Fair — last year — and I promised him my vote. He got it. Let’s hope it counts.
11:25 PM ▸ What’s Coleman on about? Looks like he got a new haircut. Seems a bit premature for him to be up addressing his supporters though. Ugh. This Senate race is going to be long and painful.
11:16 PM ▸ Wow, a president who’s not just thinking of how much he can take from the next four years, but how much he — and we — can give to the world a century from now. Vision. We have it again.
11:09 PM ▸ “This victory alone is not the change we seek. It is only the chance to make that change.”
11:03 PM ▸ See, everyone? Obama = puppies! Yay!!!
11:01 PM ▸ I know whether you’ve won or lost plays a factor in this, but when McCain mentioned Obama, his supporters booed. When Obama mentioned McCain, his supporters cheered.
10:41 PM ▸ OK, I’m back, two Baileys-es later. Let’s see how the lesser races go. Come on, Al Franken!
9:40 PM ▸ Congratulations to Joe Biden… for winning re-election to his Senate seat. I’m going to put the computer away for a while and toast President Obama with a tasty beverage!
9:36 PM ▸ OK, we can stop talking about how a year ago everyone was thinking this would be a Clinton vs. Giuliani race. I do like how they’re tiptoeing around the obvious fact that Obama has California and the other West Coast states. None of what they are saying is even remotely relevant! It’s over!
9:19 PM ▸ Yes, folks, it’s over. The networks can’t “officially” call states where the polls are still open, but there’s no real doubt that Obama will carry California, Oregon, Washington and Hawaii. If he’s already got 207, plus California’s 55, that’ll put him at 262. (To say nothing of the other three states I just mentioned.) Eight more votes from any of the other states, which he’s certain to get, and it’s over.
9:16 PM ▸ Wow, John Murtha got re-elected despite his missteps in referring to his own constituents as racists and then, in his semi-apology, as rednecks.
9:04 PM ▸ Now Nate Silver joins me in calling it for Obama, as of 8:46 PM.
8:54 PM ▸ Taking a break now for the kids’ nighttime rituals, slightly abridged.
8:52 PM ▸ Tim Russert, we miss you and your whiteboard.
8:47 PM ▸ Hmmm… as this map is starting to fill in, it’s looking like a Civil War map. All of the northern states are blue; all of the southern states are red. Let’s see how Virginia and North Carolina go. It would certainly be symbolic if the old Confederate capital, at least, went for Obama.
8:33 PM ▸ Combining the call of Ohio with FiveThirtyEight.com‘s “safe” votes, we’re at 279. Folks, I think it’s over!
8:31 PM ▸ Virginia’s coming back in line… I’m giddy! 200-to-85 right now.
8:27 PM ▸ Obama wins Ohio! Big news!!! Jw can breathe a sigh of relief.
8:26 PM ▸ Now that Minnesota’s in for Obama, I’m waiting to hear about the Senate race and the 6th District House race. I also want to hear how the Minneapolis school board results turned out. If you want to homeschool, fine. But, if you ask me, a homeschooler simply doesn’t belong on the school board!
8:17 PM ▸ Ugh. The “I’m a PC” ads sucked bad enough before they were all shot on $20 Walmart webcams.
8:15 PM ▸ FiveThirtyEight.com is now saying Obama has 259 “safe” electoral votes. If they’re right, he only needs to snag 11 more out of the toss-up pool and it’s over.
8:14 PM ▸ I now have a mispronunciation that I hate more than “nucular.” It is not pronounced “pundint”!!!!
8:05 PM ▸ Grant Park, Chicago: They got their tickets “in” the Internet? Don’t you mean the “Inter-Tubes”?
8:04 PM ▸ I am now seeing the benefit of HD. There’s all kinds of extra data crammed into the sides of the screen. I guess we’ll be sticking with the broadcast networks now. (Too cheap for digital cable.)
8:03 PM ▸ Painting a map on the ice rink at Rockefeller Center. Sweet.
8:01 PM ▸ W00t! Obama wins Minnesota. Arizona TOO CLOSE TO CALL. Hmmm….
7:59 PM ▸ Flipped to KARE-11. Not that I really need to see these guys in High Definition. I thought the one dude was Newt Gingrich at first. *shudder*
7:55 PM ▸ MSNBC, you’re losing me. If I gave a crap what Tom Delay thinks, I’d watch Fox News.
7:53 PM ▸ Chatting with SLP over Facebook even though we’re sitting in the same room. This is ridiculous.
7:45 PM ▸ I wonder if the Republican Party is on track to be relegated to the margins the way the Democrats were in the ’50s, such as in 1952 and 1956 when (both times) Adlai Stevenson only won a small band of Deep South states against the Eisenhower juggernaut. I don’t think that transition is happening this year, but based on how things go over the next four years, the 2012 electoral map might look like a mirror image of the 1952 map.
7:38 PM ▸ I don’t think this is going to move as smoothly as I had initially hoped. Virginia was looking solid Obama over the last few days on FiveThirtyEight.com.
7:31 PM ▸ Virginia… ouch. Too close to call, but McCain is way ahead with roughly a third of the precincts reporting… THIS JUST IN — Elizabeth Dole lost her seat. That was a pretty nasty campaign.
7:27 PM ▸ OK, it’s on. We’re watching MSNBC. Ann Curry was just talking about white voters in North Carolina who said race was a factor. (I think it was North Carolina. Some southern state, at any rate.) Apparently 24% of white voters in that state said race was a factor, and among them 30% “still” (that’s a quote) voted for Obama. Isn’t it possible that race was a factor for some of them in a positive way? I’m white, and I think it’s about damn time we have a black president too!
7:25 PM ▸ Is it over? I just got home. I heard on NPR in the car that Obama took Pennsylvania. Big news. McCain has a steep climb to recover from this position.
5:23 PM ▸ This will probably be my only update-by-iPhone. The on-screen keyboard is just not designed for coding HTML. Anyway, I’m at the mall with the kids, having Chicken McNuggets shoved in my face. Not by choice. Oh well… the cashier at McDonalds gave me a free milk when she saw my Obama button. Let the redistribution begin!
2:27 PM ▸ OK, time to go think about something else for a while. I’ll be back when I just can’t stand it anymore.
1:56 PM ▸ Tick… tock… tick… tock…. Is it over yet?
11:54 AM ▸ My Voting Story. Since I’m already starting to hear tales of woe from friends and acquaintances in other states, I felt like I should share my own story. Gloat, if you will. We decided to vote after the kids were off at school, so we ended up walking to our polling place (the Methodist church at the end of our block) around 9:15 AM. We talked to some neighbors along the way, who had already voted, and they mentioned that there had been a bit of a line earlier as people voted before work, but now things had tapered off.
We walked right in, no line, signed in, walked over to the ballot table, took our ballots to the little folding (and flimsy) voting carrels, marked ’em up, took them to the Tabulatron 2000™ (or whatever it’s called), fed them in, and that was it. Got our stickers and walked out. OK, the Tabulatron spit out my ballot at first so I had to give it a second try, but then it worked. I even distinctly heard the sound of the ballot dropping, intact, to the bottom of the big metal box inside, so I’m fairly sure it was not shredded.
Now, lest you get the impression that the electorate is staying home around here, that is not the case. The place was humming with people. It just happened that the capacity of the polling place was precisely matched to the number of people coming in to vote at that time. I could almost hear a Raymond Scott tune playing in the background.
Go Minneapolis!
11:39 AM ▸ Popcorn has been requested. Here you go…
11:23 AM ▸ He hasn’t even won yet and they’re already renaming buildings after him.
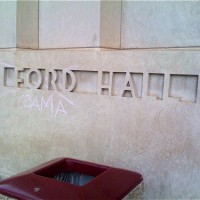
11:11 AM ▸ I managed to work for an hour. Now it’s time to get some lunch and think about the election some more. And post some asinine status updates on Facebook.
10:11 AM ▸ Currently on the U of M campus. Election buzz is tangible. Lots of Obama love. Also clean water amendment. Yay, sales tax! (I voted for that, too.)
9:25 AM ▸ The deed is done.
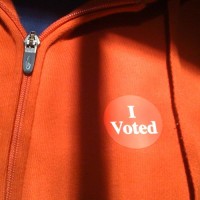
6:34 AM ▸ Let’s get things started by dropping in the MSNBC election dashboard. This will serve as the Al Michaels (or, if you’re old skool, Pat Summerall) to my John Madden.